The Role of Object-Relational Mapping (ORM) in Modern Application Development
Introduction to ORM Tools
Object-Relational Mapping (ORM) is a programming technique used to convert data between incompatible systems. ORMs allow developers to interact with a database using the programming language’s objects, rather than writing complex SQL queries. This simplifies CRUD operations and provides an abstraction layer over the database, making development easier and more maintainable.
In this article, we’ll compare 7 popular ORM tools:
- JPA (Java Persistence API)
- EF (Entity Framework)
- Sequelize (Node.js ORM)
- TypeORM (Node.js ORM)
- Doctrine (PHP)
- Django ORM (Python)
- Objection.js (Node.js)
Object-Relational Mapping (ORM) Usage in Modern Development
One of the primary reasons developers turn to Object-Relational Mapping (ORM) tools is the significant productivity boost they provide. ORMs allow developers to interact with databases using their programming language’s syntax rather than raw SQL. This makes database operations more intuitive, especially for those who may not be experts in SQL. It also speeds up the development process by abstracting away the need to write repetitive queries. ORM tools also help ensure consistency and maintainability in the codebase by using a unified data model across the application. This avoids the need to embed SQL queries throughout, which keeps the code cleaner and easier to manage.
Beyond productivity, security is another critical reason to use an ORM. By abstracting SQL queries, ORMs protect developers from common vulnerabilities like SQL injection attacks. Since ORM frameworks typically use parameterized queries behind the scenes, they automatically safeguard applications from malicious user input. This can significantly reduce the risk of vulnerabilities, as developers no longer have to sanitize SQL inputs manually.
ORMs also provide built-in methods for managing database migrations, allowing developers to control and version schema changes. This prevents database inconsistencies and eliminates the risk of manual SQL errors. Additionally, most ORMs support lazy and eager loading, giving developers control over data retrieval strategies. This helps balance performance with resource efficiency, especially when handling large datasets and complex relationships.
ORMs offer an efficient, secure, and maintainable way to manage database operations, making them indispensable for developers building modern applications. By reducing the complexity of database interactions, ORMs allow developers to focus more on business logic. They also help ensure that applications remain safe and efficient.
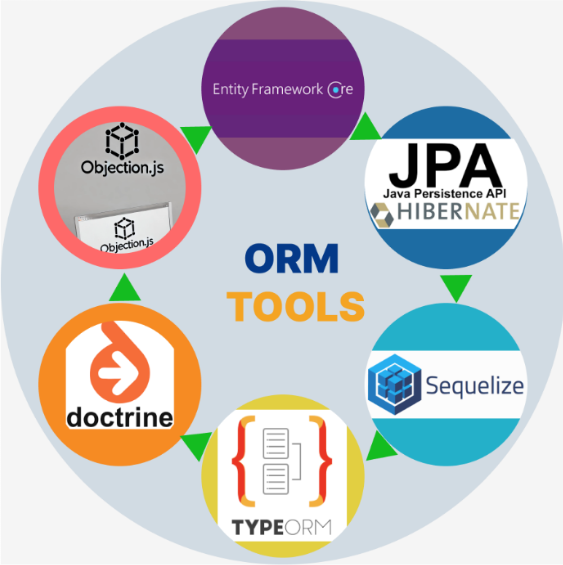
1. Java Persistence API (JPA)
The Java Persistence API (JPA) is a core tool for developers working in the Java ecosystem to manage relational data. JPA provides a set of APIs that act as a bridge between Java objects and database tables. It’s not a framework by itself but serves as a specification that frameworks like Hibernate and EclipseLink implement. This means that instead of writing long SQL queries, you can use Java annotations like @Entity or @Table. These annotations map your objects to the database tables, making the data management process more intuitive.
JPA simplifies handling complex relationships, such as one-to-many or many-to-many associations between entities. It also manages entity states with the persistence context, which can cache operations and improve performance. Additionally, JPA offers JPQL (Java Persistence Query Language), a SQL-like syntax tailored for Java objects, giving developers a powerful way to query databases without diving into raw SQL.
JPA stands out as an established standard in Java, offering flexible approaches. Whether you’re building your models first or starting from an existing database, JPA can adapt to your workflow. However, the learning curve can be steep, particularly when integrated with Hibernate. Additionally, the abstraction can occasionally introduce performance overhead, making JPA less suited for high-performance, low-level operations.
2. Entity Framework (EF)
For developers in the .NET ecosystem, Entity Framework (EF) is the ORM of choice. It allows you to interact with your database using C# objects, significantly reducing the need to write SQL queries manually. One of the standout features of EF is its tight integration with LINQ (Language-Integrated Query), which lets developers write queries using C# syntax that is both powerful and readable.
EF is versatile, offering both code-first and database-first approaches. This allows you to either define your data models and generate the database schema from them or start with an existing database and generate your models from it. Additionally, EF comes with robust migration tools that make it easy to manage schema changes over time. You can also control data fetching with lazy or eager loading to optimize performance.
While EF provides deep integration with .NET and excellent tools for working with complex data models, it can be more challenging for beginners to master. Moreover, its performance, though generally strong, can lag behind raw SQL when working with very large datasets.
3. Sequelize
Sequelize is one of the most widely ORMs in the Node.js ecosystem. Thanks to its flexibility and ease of use, Sequelize helps developers interact with SQL databases such as PostgreSQL, MySQL, SQLite, and MariaDB without having to write SQL queries manually. Instead, Sequelize allows you to define your data models using JavaScript or TypeScript, making it a natural fit for developers in the Node.js environment.
Sequelize supports defining complex relationships between models (such as one-to-many or many-to-many) and comes equipped with features like migrations and seeding, making schema changes and initializing data straightforward. It also offers query builders that allow you to construct complex database queries in JavaScript, making it easy to retrieve data without diving into raw SQL.
However, while Sequelize is user-friendly and backed by a large community, it lacks some of the more advanced features of older ORMs like JPA and EF. Additionally, when working with very large datasets, performance can be a concern due to the added abstraction layer.
4. TypeORM
Another powerful option for Node.js is TypeORM, a more feature-rich ORM compared to Sequelize, particularly when working with TypeScript. It brings advanced ORM features often seen in Java and .NET ecosystems into Node.js, such as support for both Active Record and Data Mapper patterns. These features allow you to organize your data models and access them in flexible ways.
TypeORM offers excellent support for various SQL databases like PostgreSQL, MySQL, and SQLite, making it versatile across different database engines. Its full TypeScript support makes it a great fit for developers who prefer static typing and compile-time error checking. Like other modern ORMs, TypeORM supports lazy and eager loading, giving developers control over how and when data is loaded from the database.
While TypeORM is robust and feature-rich, it comes with a more complex setup than Sequelize, and the learning curve can be steeper, especially for developers new to TypeScript or ORM concepts in general. However, TypeORM’s extensive feature set is a significant advantage for those building more complex applications.
5. Doctrine (PHP)
In the PHP world, Doctrine stands out as the ORM choice, widely adopted in frameworks like Symfony, though it’s flexible enough to be used in standalone PHP applications as well. Doctrine’s biggest strength lies in its ability to map objects to database tables using different formats, including annotations, YAML, or XML. This provides developers with the flexibility to choose the approach that best fits their project.
Doctrine also supports lazy and eager loading, giving developers control over when and how related entities are fetched. Its built-in migration system helps manage schema changes over time, a must-have for applications that evolve constantly.
However, while Doctrine offers a flexible and powerful ORM system, it may come with some performance drawbacks, especially for very complex queries, due to the added overhead of abstraction. Moreover, Doctrine can take time to master, particularly for developers who are new to PHP or ORMs in general.
6. Django ORM (Python)
For Python developers using the Django framework, the Django ORM is an integral part of the package, allowing developers to manage their database models without having to worry about SQL. Django ORM provides a high-level abstraction for interacting with relational databases, mapping database tables to Python classes automatically, making it a seamless experience for web developers.
One of the major strengths of Django ORM is its ability to automatically create and manage the database schema based on the models you define. It also offers built-in migrations, making it easy to version control your database changes. Querying data is made intuitive through QuerySets, which allows you to filter and retrieve data with simple, chainable syntax.
Although Django ORM is easy to use and well-integrated with the framework, it comes with some limitations. It only supports a handful of databases like PostgreSQL, MySQL, and SQLite, and for highly complex queries, you may need to drop down to raw SQL for optimal performance.
7. Objection.js (Node.js)
Objection.js is a lightweight ORM for Node.js, built on top of the Knex.js query builder. Unlike traditional ORMs that abstract SQL away entirely, Objection.js strikes a balance by allowing you to write SQL queries directly when necessary, while still offering the ability to define models and relationships through JavaScript or TypeScript classes.
Objection.js supports complex relationships, such as one-to-many and many-to-many, and integrates well with schema validation libraries like Joi or Ajv, providing strong validation mechanisms out of the box. It also features graph inserts, which make it easier to manage complex nested data structures in a single operation.
While Objection.js gives you the flexibility to write raw SQL when needed, it may not be the best choice for those who want a fully abstracted ORM experience. It is less widely adopted than Sequelize or TypeORM, though it remains actively developed and is a solid choice for those seeking more direct control over their SQL operations.
Differences Table
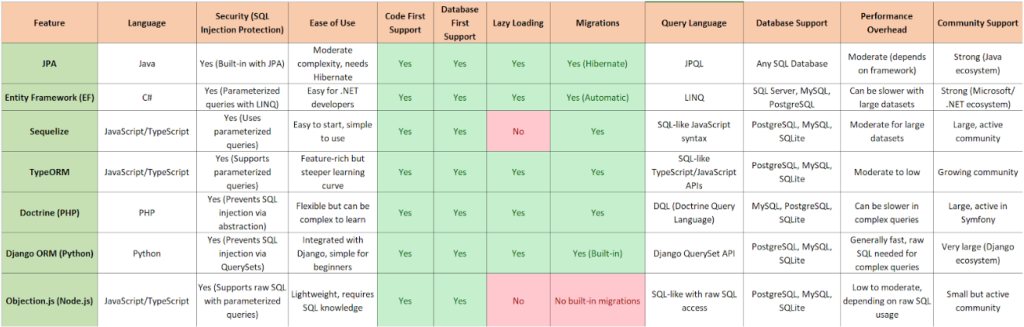
ORMs and SQL Injection Prevention
One of the significant security benefits of using an Object-Relational Mapping (ORM) tool is its built-in protection against SQL injection attacks. In traditional SQL, when user inputs are directly embedded into queries, malicious users can inject harmful SQL commands. ORMs mitigate this risk by using parameterized queries or prepared statements.
Instead of concatenating user inputs directly into SQL strings, ORMs automatically bind inputs as parameters, ensuring that they are treated strictly as data, not executable code. This protects applications from unauthorized database manipulation, making ORM a crucial tool in developing secure, database-driven applications.
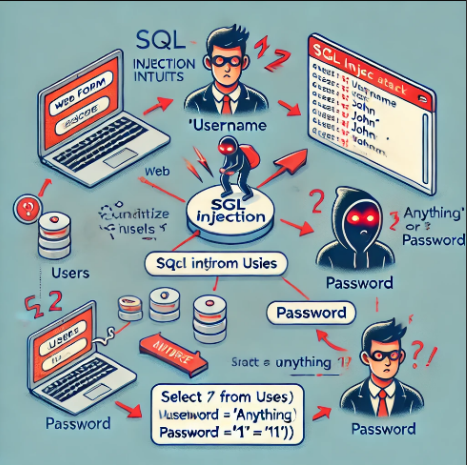
Conclusion
When choosing an Object-Relational Mapping (ORM) tool, it’s important to consider the ecosystem you’re working in, the complexity of your data model, and how much control you need over database queries. JPA and Entity Framework are mature, full-featured ORMs ideal for large-scale enterprise applications in Java and .NET ecosystems. Sequelize and TypeORM are solid choices for Node.js developers, with TypeORM offering more advanced features and tighter TypeScript integration. Doctrine provides a robust and flexible ORM solution for PHP applications, while Django ORM simplifies the database interaction for Python developers using the Django framework. For those who need more direct access to SQL with minimal overhead, Objection.js is a great option for Node.js projects.
At Mysoly, we prioritize both performance and security in our software development practices. By leveraging Object-Relational Mapping (ORM) tools in our projects, we not only streamline database interactions but also ensure that our code is safeguarded against risks like SQL injection. We believe that by using ORMs, we can deliver secure, scalable, and maintainable solutions to meet the needs of our clients.
Mysoly | Your partner in digital!
bilal cangal serkan kilic